Call Aria Automation REST API from a Aria Automation Orchestrator Workflow
In this post I show you how you can call the Aria Automation REST API from a Aria Automation Orchestrator Action.
Prerequisites:
Create an Action with following inputs and Return type:
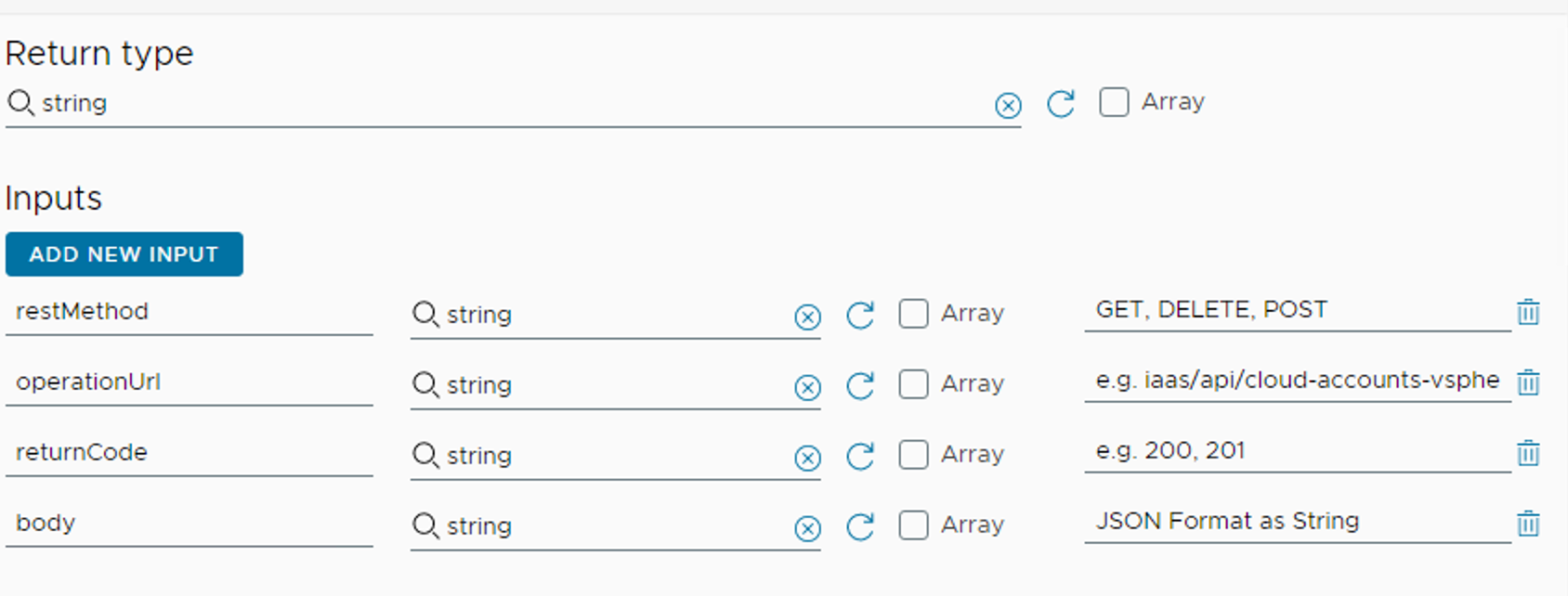
The Return value is a JSON string. If you want get the values of the JSON string you must convert it to an JSON Object with JSON.stringify(return value).
Add the following Script into the Action Script canvas:
var vraHost = System.getModule("com.vmware.pso.util").getConfigElementAttributeValue("PSO/vRA", "Global Config", "defaultvRA");
var apiVersion = System.getModule("com.vmware.pso.util").getConfigElementAttributeValue("PSO/vRA", "Global Config", "apiVersion");
var restClient = vraHost.createRestClient(); // Create vRA REST Client
if (!apiVersion) {
var request = restClient.createRequest(restMethod.toUpperCase(), encodeURI(operationUrl));
var response = restClient.execute(request)
if (response.statusCode != "200") throw "REST request failed with staus code " + response.statusCode + "\r\nResponse is: " + response.contentAsString
apiVersions = JSON.parse(response.contentAsString)
apiVersion = apiVersions.latestApiVersion
}
if (vraHost) {
System.log("SWITCH TO DEBUG LOG TO SEE ALL LOGs")
System.debug("Used APi Version: " + apiVersion)
var content = []
if (operationUrl.indexOf("?") != -1) {
operationUrl = encodeURI(operationUrl + "&apiVersion=" + apiVersion)
} else {
operationUrl = encodeURI(operationUrl + "?apiVersion=" + apiVersion)
}
//operationUrl = decodeURI(operationUrl)
System.debug("++++++++++++++++++ STARTING Aria Automation REST Call +++++++++++++++++++++++++")
System.debug("Requested URL: " + operationUrl)
if (body != null && body != "") {
System.debug("Payload for REST Call: " + JSON.stringify(JSON.parse(body), null, 2))
var request = restClient.createRequest(restMethod.toUpperCase(), operationUrl, body);
} else {
var request = restClient.createRequest(restMethod.toUpperCase(), operationUrl);
}
var response = restClient.execute(request)
if (response.statusCode != returnCode) throw "REST request failed with staus code " + response.statusCode + "\r\nResponse is: " + response.contentAsString
System.debug("Status Code: " + response.statusCode)
if (response.contentAsString) {
var res = JSON.parse(response.contentAsString)
if (res.content) {
if (res.pageable && res.numberOfElements == 20 && operationUrl.indexOf("page") == -1) {
if (res.totalPages) {
var totalPages = res.totalPages
if (totalPages > 1) {
System.debug("Is Pagable")
content = myPage(totalPages, restClient, content)
var myContent = {}
myContent.content = content
}
}
} else {
System.debug("Aria Automation REST Call Response: " + JSON.stringify(JSON.parse(response.contentAsString), null, 4))
System.debug("++++++++++++++++++ END Aria Automation REST Call WITHOUT Paging +++++++++++++++++++++++++")
return response.contentAsString;
}
} else {
System.debug("Aria Automation REST Call Response: " + JSON.stringify(JSON.parse(response.contentAsString), null, 4))
System.debug("++++++++++++++++++ END Aria Automation REST Call WITH ONLY ONE ELEMENT +++++++++++++++++++++++++")
return response.contentAsString;
}
}
} else {
throw ("No vRA Host in the Configuration Elemnent: 'PSO/vRA/Global Config/vraHost' configured, Please configure it.")
}
if (myContent != null && myContent.content.length != 0) {
System.debug("Aria Automation REST Call Response: " + JSON.stringify(myContent, null, 4))
System.debug("++++++++++++++++++ END Aria Automation REST Call WITH Paging (" + myContent.content.length + " Elements found.) +++++++++++++++++++++++++")
return JSON.stringify(myContent, null, 4)
}
function myPage(numberOfPages, restClient, content) {
var numberOfPages = numberOfPages - 1
while (numberOfPages >= 0) {
var myOperrationUrl = operationUrl + "&page=" + numberOfPages
myOperationUrl = decodeURI(myOperrationUrl)
System.debug("Paging URLs: " + myOperrationUrl)
var request = restClient.createRequest(restMethod.toUpperCase(), encodeURI(myOperationUrl));
var response = restClient.execute(request);
if (response.statusCode != returnCode) throw "REST request failed with staus code " + response.statusCode + "\r\nResponse is: " + response.contentAsString
var response = response.contentAsString
response = JSON.parse(response)
if (response.content) {
content = content.concat(response.content)
}
numberOfPages = numberOfPages - 1
}
return content
}